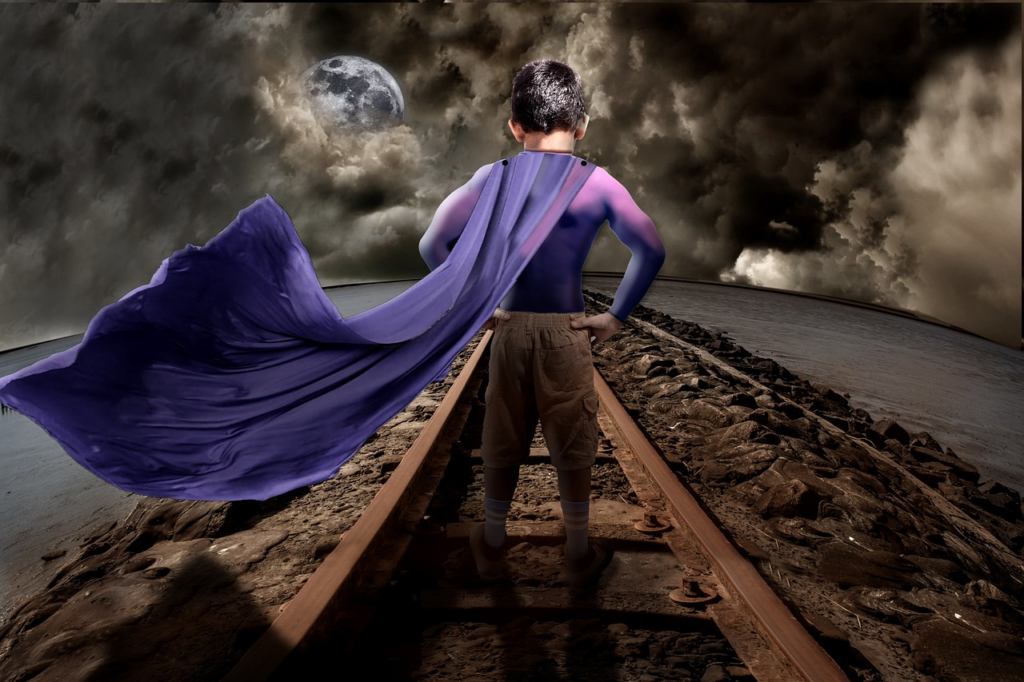
Introduction
Error handling is a critical aspect of programming, regardless of the language being used. Writing effective error-handling code can be challenging, whether your language supports exception handling or not. It requires careful consideration of the types of exceptions that may occur and how to handle them appropriately. In this guide, we will delve into the different categories of exceptions and provide practical strategies for handling them in your code.
Understanding Exception Categories
When it comes to exception handling, it’s important to classify exceptions into distinct categories. By doing so, you can determine the appropriate course of action for each type of exception. Let’s explore the four main categories: fatal, boneheaded, vexing, and exogenous exceptions.
1. Fatal Exceptions
Fatal exceptions are those that are beyond your control. They occur when a process is deeply flawed and cannot be salvaged. Examples of fatal exceptions include out of memory errors or thread abortion. Since there is no way to recover from these exceptions, it is generally best to let your finally blocks run and hope for the best. In some cases, failing fast and preventing the execution of finally blocks may be necessary to avoid further complications.
2. Boneheaded Exceptions
Boneheaded exceptions are entirely your fault as a programmer. These exceptions occur due to coding errors that could have been easily prevented. Instead of catching boneheaded exceptions, it is recommended to write code in a way that eliminates the possibility of such exceptions. For example, validating input parameters, avoiding null arguments, or checking for out-of-range indexes can help prevent boneheaded exceptions from occurring.
3. Vexing Exceptions
Vexing exceptions are the result of design choices that lead to non-exceptional circumstances triggering an exception. These exceptions are often frustrating because they require constant handling. An example of a vexing exception is the Int32. Parse method in C#, which throws an exception when attempting to parse an invalid integer. To handle vexing exceptions, it is advisable to use the “Try” versions of methods that provide non-exceptional return values, such as TryParse. This way, you can avoid the need for exception handling altogether.
4. Exogenous Exceptions
Exogenous exceptions are not the result of poor design choices but rather external factors that affect your program’s execution. These exceptions arise from unpredictable events, such as file not found errors or network failures. While it may be tempting to eliminate try-catch blocks when handling exogenous exceptions, it is crucial to consider race conditions and the time gap between the check and the use of resources. Handling exogenous exceptions is necessary because they are circumstances outside of your control.
Best Practices for Exception Handling
Now that we have explored the different categories of exceptions, let’s discuss some best practices for handling exceptions in your code. These practices will help you write robust and maintainable code.
1. Avoid Catching Fatal Exceptions
As mentioned earlier, fatal exceptions are beyond your control and cannot be fixed by your code. Therefore, it is generally best to avoid catching these exceptions. Instead, focus on allowing your finally blocks to run and handle any necessary cleanup operations.
2. Prevent Boneheaded Exceptions
To prevent boneheaded exceptions, it is crucial to write code that eliminates the possibility of these errors. Take proactive measures such as validating inputs, performing appropriate type casting, and checking for out-of-range values. By addressing potential issues before they occur, you can avoid the need for exception handling altogether.
3. Minimize Vexing Exceptions
While it may not always be possible to eliminate vexing exceptions entirely, you can minimize their occurrence by using the “Try” versions of methods whenever available. These methods provide a non-exceptional return value, allowing you to handle potential issues without relying on exception handling.
4. Handle Exogenous Exceptions
Exogenous exceptions are often unpredictable and can occur despite your best efforts to avoid them. It is essential to handle these exceptions to ensure your program can gracefully recover or provide appropriate error messages to users. Consider the external factors that may impact your program’s execution and handle exceptions accordingly.
Implementing Effective Error Handling Strategies
To implement effective error handling in your code, follow these strategies:
1. Use Exception Hierarchies
Organize your exceptions into hierarchies based on their types and relationships. By doing so, you can catch exceptions at different levels and handle them appropriately. This approach allows for more granular exception handling and better code maintainability.
2. Provide Meaningful Error Messages
When an exception occurs, it is essential to provide meaningful error messages that help users understand what went wrong. Include relevant information, such as the specific error code or a description of the problem. Clear and informative error messages can significantly aid in troubleshooting and debugging.
3. Log Exceptions
Logging exceptions is crucial for diagnosing issues and monitoring the performance of your application. By logging exceptions, you can track errors and gain insights into potential patterns or recurring issues. This information can be invaluable for identifying and resolving problems in your code.
4. Graceful Degradation
When encountering exceptions, it is essential to handle them gracefully and provide fallback options whenever possible. Graceful degradation ensures that your application can continue functioning to some extent, even when unexpected errors occur. This approach can enhance the user experience and minimize disruptions.
Conclusion
Mastering error handling in programming is a crucial skill that can greatly enhance the reliability and robustness of your code. By understanding the different categories of exceptions and implementing effective error-handling strategies, you can create code that gracefully handles errors and provides a better user experience. Remember to prioritize prevention, meaningful error messages, and logging to facilitate debugging and troubleshooting. With these practices in place, you can elevate your programming skills and write code that is more resilient to exceptions.
Additional Information:
- It’s important to note that while the article emphasizes the importance of preventing boneheaded exceptions, there may be cases where catching and handling them is necessary. Use your judgment to determine the best approach based on the specific context and requirements of your code.